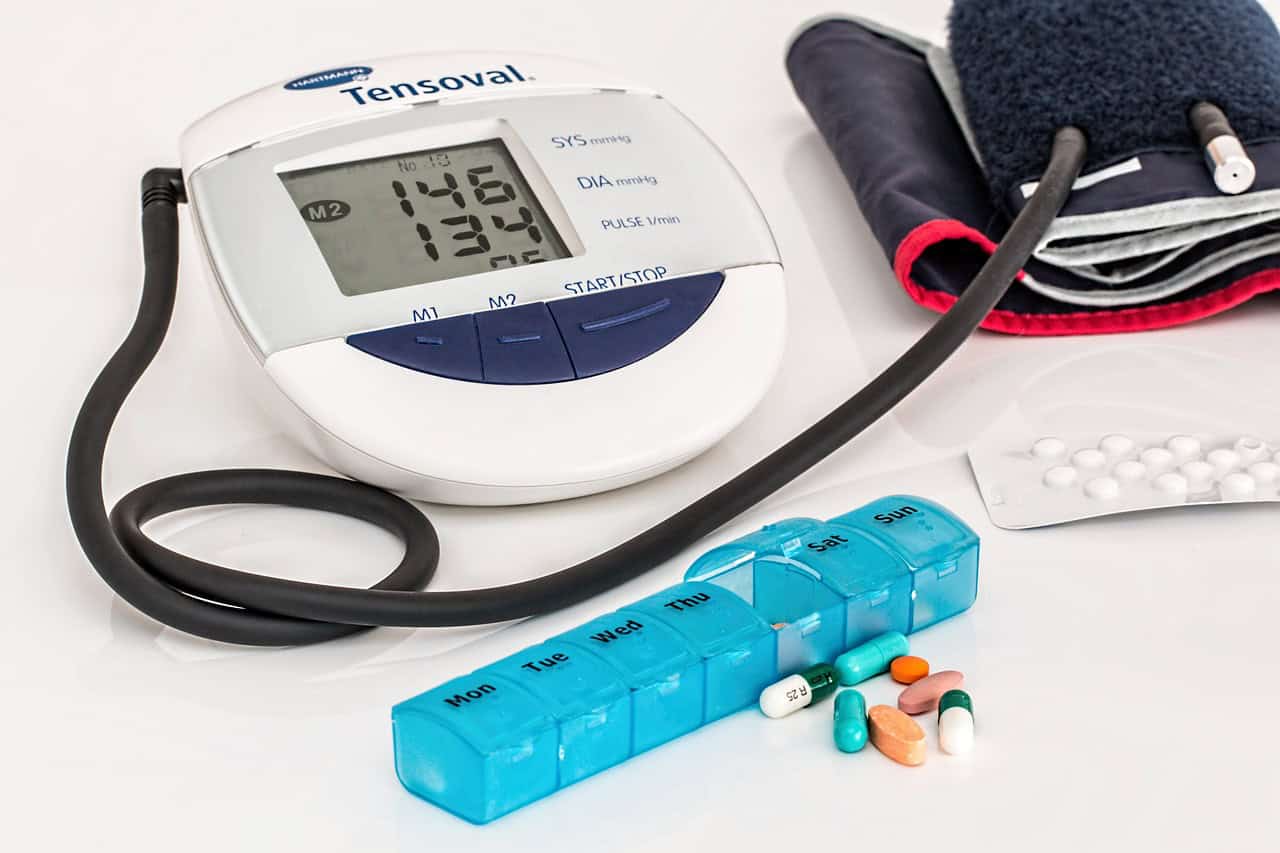
A few of the particular points on this dataset embrace:
- Main and trailing areas in string columns.
- Non-numeric values in numeric columns (e.g., ‘forty’ as a substitute of 40 within the Age column).
- Incorrect or lacking entries in columns like Blood Stress, Ldl cholesterol, and Go to Date.
The dataset will be downloaded utilizing this GitHub link.
Step-by-step information cleansing course of
Let’s dive into the steps to scrub this dataset. Under, we break down every step with corresponding Python code to remodel the dataset right into a clear and analysis-ready format.
Step 1: Load the dataset
First, we have to load the dataset utilizing Pandas:
import pandas as pd
# Load the messy information
df_healthcare_messy = pd.read_csv('path_to/healthcare_messy_data.csv')
NB: Please exchange the “path_to” with the precise location of the saved messy information in your machine.
Step 2: Strip main and trailing areas
This dataset might seemingly comprise pointless areas in string columns, which might trigger issues throughout evaluation. We take away these areas utilizing the next code:
# Strip main and trailing areas from string columns
for column in df_healthcare_messy.select_dtypes(embrace=['object']).columns:
df_healthcare_messy[column] = df_healthcare_messy[column].str.strip()
Step 3: Appropriate non-numeric values
Some columns, like Age, comprise non-numeric values that needs to be transformed:
# Appropriate the Age column
df_healthcare_messy['Age'] = df_healthcare_messy['Age'].exchange('forty', 40) # Substitute 'forty' with 40
df_healthcare_messy['Age'] = pd.to_numeric(df_healthcare_messy['Age'], errors="coerce") # Convert to numeric, coercing errors to NaN
# Fill NaN values within the Age column earlier than changing to int
df_healthcare_messy['Age'] = df_healthcare_messy['Age'].fillna(0).spherical(0).astype(int) # Take away decimals and convert to int
Equally, for different numeric columns like Blood Stress and Ldl cholesterol:
# Appropriate the Blood Stress column
df_healthcare_messy['Blood Pressure'] = df_healthcare_messy['Blood Pressure'].exchange('NaN', np.nan)
# Appropriate the Ldl cholesterol column
df_healthcare_messy['Cholesterol'] = pd.to_numeric(df_healthcare_messy['Cholesterol'], errors="coerce") # Convert to numeric, coercing errors to NaN
# Fill NaN values within the Ldl cholesterol column earlier than changing to int
df_healthcare_messy['Cholesterol'] = df_healthcare_messy['Cholesterol'].fillna(0).spherical(0).astype(int) # Take away decimals and convert to int
Step 4: Standardise date columns
Dates are sometimes saved in numerous codecs. Standardising them ensures consistency:
# Standardise the Go to Date column
df_healthcare_messy['Visit Date'] = pd.to_datetime(df_healthcare_messy['Visit Date'], errors="coerce")
Step 5: Deal with lacking values
Lacking information is a standard problem in datasets. We will deal with lacking values by filling them with applicable defaults:
# Fill NaN values with a default worth or technique
df_healthcare_messy['Age'].fillna(df_healthcare_messy['Age'].imply(), inplace=True)
df_healthcare_messy['Cholesterol'].fillna(df_healthcare_messy['Cholesterol'].imply(), inplace=True)
# Fill NaN values for categorical columns with a placeholder or most frequent worth
df_healthcare_messy['Gender'].fillna('Unknown', inplace=True)
df_healthcare_messy['Condition'].fillna(df_healthcare_messy['Condition'].mode()[0], inplace=True)
df_healthcare_messy['Medication'].fillna(df_healthcare_messy['Medication'].mode()[0], inplace=True)
df_healthcare_messy['Blood Pressure'].fillna('120/80', inplace=True)
df_healthcare_messy['Email'].fillna('no_email@instance.com', inplace=True)
df_healthcare_messy['Phone Number'].fillna('000-000-0000', inplace=True)
Step 6: Save the cleaned dataset
Lastly, we save the cleaned dataset to a brand new CSV file for future use:
# Save the cleaned information to a brand new CSV file
df_healthcare_messy.to_csv('path_to/cleaned_healthcare_messy_data.csv', index=False)
NB: Please exchange the “path_to” with the precise location the place you desire to the cleaned dataset to be saved in your machine.
Abstract of cleansing steps
To summarise, the next steps had been taken to scrub the dataset:
- Loaded the dataset utilizing Pandas.
- Stripped main and trailing areas from string columns.
- Corrected non-numeric values in numeric columns.
- Standardised date codecs within the Go to Date column.
- Dealt with lacking values by filling them with applicable defaults or most frequent values.
- Saved the cleaned dataset for future evaluation.
The cleaned dataset will be obtain utilizing this GitHub link.
Under is only a snippet of the cleaned dataset:
Affected person Title | Age | Gender | Situation | Medicine | Go to Date | Blood Stress | Ldl cholesterol | E mail | Telephone Quantity |
---|---|---|---|---|---|---|---|---|---|
david lee | 25 | Different | Coronary heart Illness | METFORMIN | 2020-01-15 | 140/90 | 200 | identify@hospital.org | 555-555-5555 |
emily davis | 0 | Male | Diabetes | NONE | 120/80 | 200 | no_email@instance.com | 000-000-0000 | |
laura martinez | 35 | Different | Bronchial asthma | METFORMIN | 110/70 | 160 | contact@area.com | 000-000-0000 | |
michael wilson | 0 | Male | Diabetes | ALBUTEROL | 2020-01-15 | 110/70 | 0 | identify@hospital.org | 555-555-5555 |
david lee | 0 | Feminine | Bronchial asthma | NONE | 110/70 | 180 | no_email@instance.com | ||
mary clark | 0 | Male | Hypertension | METFORMIN | 140/90 | 180 | no_email@instance.com | 000-000-0000 | |
robert brown | 0 | Male | Hypertension | LISINOPRIL | 120/80 | 0 | identify@hospital.org | 000-000-0000 | |
david lee | 60 | Different | Bronchial asthma | NONE | 120/80 | 0 | identify@hospital.org | 000-000-0000 |
Conclusion
Cleansing a dataset is an important step earlier than any data analysis. On this tutorial, we’ve walked via a scientific strategy to deal with frequent information points corresponding to lacking values, inconsistent codecs, and incorrect information entries within the healthcare information.
With the dataset now clear, you’re able to carry out correct and significant analyses. Keep in mind, a clear dataset is a basis for dependable insights!
Be at liberty to regulate the code snippets based on your particular dataset, and pleased coding!
Trending Merchandise